Goals and Objectives
We have specified the hardware and software requirements for the Base-station in Section
Components of Project 4.
Please, recall that the base-station is comprised of the following:
Figure 1: Workstation of the base-station showing the three tracked Roomba robots. It also shows the transmitter module.
Figure 2: The tracker camera with the workstation. The image was taken before plugging in the the trajectory transmitter. Recall that the base-station is comprised of a workstation, a Kinect sensor, and a micro-controller based transmitter.
All these modules are physically connected together and communicate over USB cables.
Note that the workstation is mainly responsible for computing the locations of the tracked Roomba robots. In Section Kinect Tracker we will describe that in detail.
These three modules communicate in a very specific manner -- in a sequential one direction manner only. The sequence is ordered as follows:
- a Kinect camera,
- a workstation to process depth data from the Kinect sensor,
- a micro-controller based wireless transmitter to transmit required speeds and radii to the satellite station.
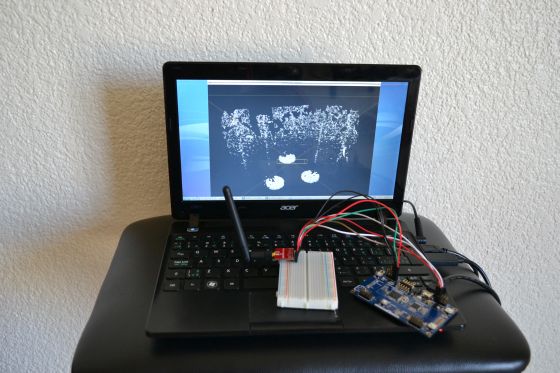
Figure 1: Workstation of the base-station showing the three tracked Roomba robots. It also shows the transmitter module.
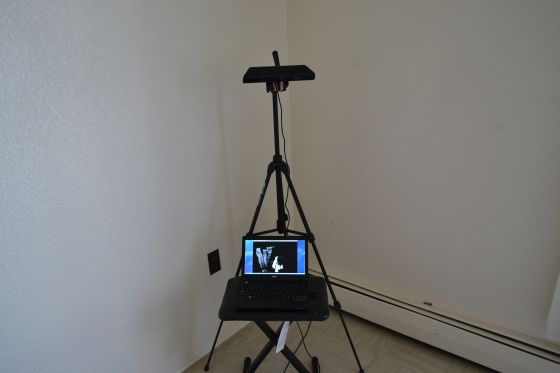
Figure 2: The tracker camera with the workstation. The image was taken before plugging in the the trajectory transmitter. Recall that the base-station is comprised of a workstation, a Kinect sensor, and a micro-controller based transmitter.
All these modules are physically connected together and communicate over USB cables.
Note that the workstation is mainly responsible for computing the locations of the tracked Roomba robots. In Section Kinect Tracker we will describe that in detail.
These three modules communicate in a very specific manner -- in a sequential one direction manner only. The sequence is ordered as follows:
- The workstation first reads the depth values from the Kinect sensor. It then computes the current position and then if necessary computes any adjustment in speeds and radii for the satellite stations.
- The workstation then transmits these values to the transmitter by a serial port.
- The transmitter then transmits these values to the satellite stations by radio.