Scheduling
In this page we will describe how the RTOS determines the next task to run. It can
handle two very important kind of tasks. They are:
This RTOS implements fixed priority pre-emptive scheduling. Scheduling takes place:
Figure 1:Kernel main loop. User tasks are scheduled by the kernel_dispatch function depending on the requests made to the kernel that gets handled in the kernel_handle_request function.
- periodic tasks, and
- one-shot tasks.
This RTOS implements fixed priority pre-emptive scheduling. Scheduling takes place:
- at an RTOS tick, and when
- a task voluntarily gives up the CPU,
- a system task is created,
- a system or an rr task waits for an event,
- a task (usually, an ISR) generates a signal for an event, and
- a task (usually a system or an rr task) finishes.
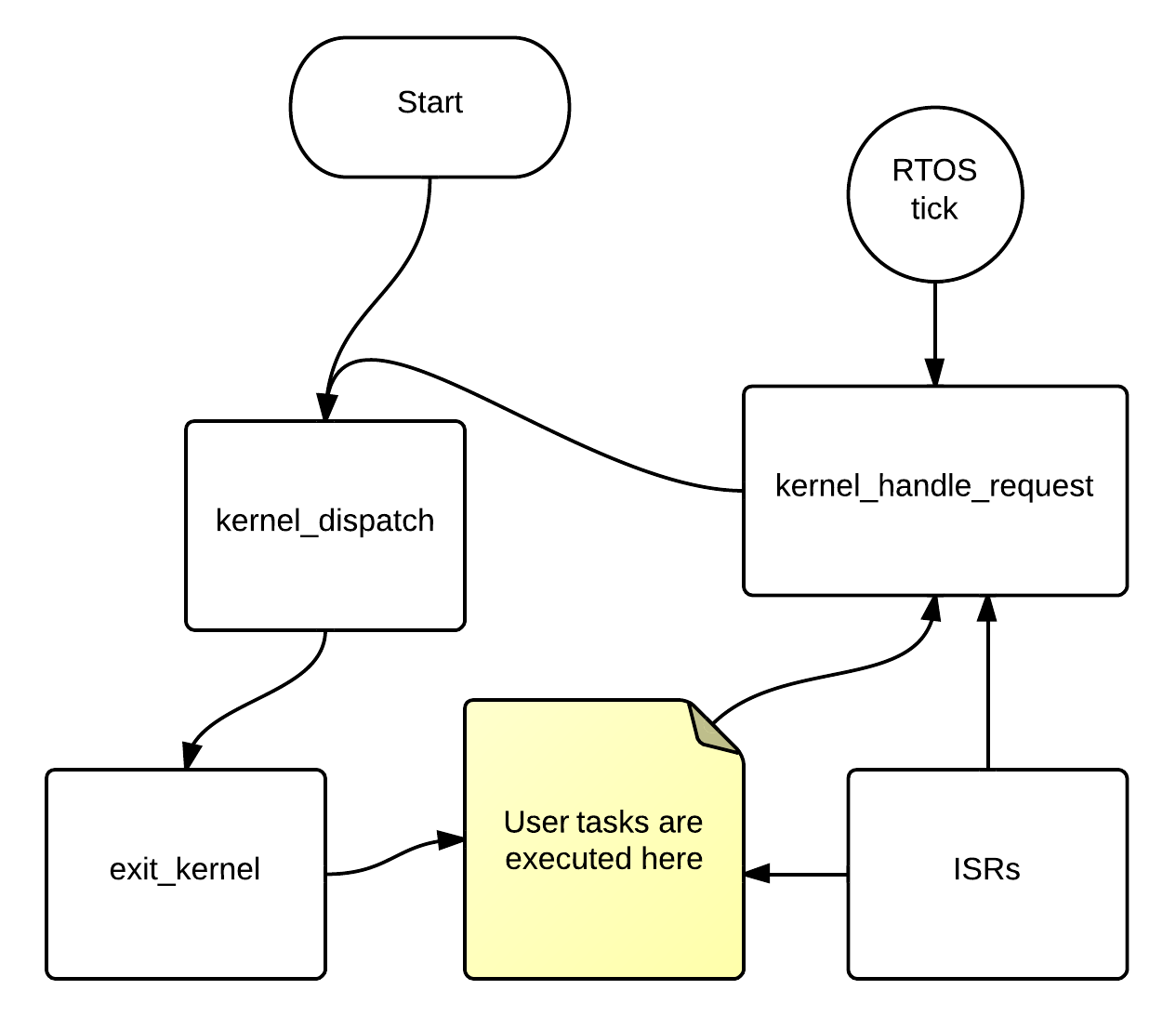
Figure 1:Kernel main loop. User tasks are scheduled by the kernel_dispatch function depending on the requests made to the kernel that gets handled in the kernel_handle_request function.
static void kernel_main_loop() { while(1){ kernel_dispatch(); exit_kernel(); kernel_handle_request(); } }The listing above shows the kernel_main_loop function. A context switch to a user task chosen by the scheduler takes place at the exit_kernel function. We will now explain what happens in the kernel handle request and kernel dispatch blocks.